The while Loop. If you review the scripts you have been writing over these past few months, you likely notice blocks of code of that look very similar except for small variations.
Programming languages offer a solution that can siginficantly reduce the amount of code you are writing. It comes in the form of a statement known as the while loop.
Task.
Download the file WhileLoop.html and complete the onclick event handler function for each question.
The String Object. You've examined the resources of JavaScript's Number and Math Objects. Mastering the property and methods of the String Object is key to great coding skills and this activity will get you started in this direction.
Task.
- Copy MathDecisions.html to StringObject.html and adjust the <title> tag.
- Click on the image to the right and duplicate the contents. The Tutorial link is
http://www.w3schools.com/js/js_strings.asp
. The Reference link is: http://www.w3schools.com/jsref/jsref_obj_string.asp
.
The Math Object. Save NumberDecisions.html as MathDecisions.html and modify the questions with the text below that capitalize on the resources (properties and methods) of the JavaScript's Math Object.
- Determine the area of a circle with radius `|x|` cm.
- Determine the volume of a sphere with radius `|z|` cm.
- Determine if `x <= pi < z`.
- Determine the length of the diagonal of a square with side length, `|y|` m.
- Determine the side length of a square with diagonal length, `|z|` m.
- Determine the length of the body diagonal of a cube of edge length, `|x|` cm.
- Determine the edge length of a cube with body diagonal length, `|z|` m.
- Display a random number on the interval, `[0, 1)`.
- Display a random number on the interval, `[0, 8)`.
- Display a random number on the interval, `[16, 24)`.
- Display a random number on the interval, `[0, |y|)`.
- if `|x|<=|y|`, display a random number on the interval, `[|x|`,`|y|)` otherwise, over the interval, `[|y|`,`|x|)` .
Decisions 2: The if..else Statement: Number and Math Decisions
- Ensure your Shell.html template is MathJax-enabled. Test it. Once confirmed, copy Shell.html to NumberDecisions.html.
- Click on the graphic to the right for a larger version of the image.
- Add three ASCIIMathML-labelled, text input boxes, `x`:, `y`:, and `z`:, id them, and provide default number values for each.
- Provide id's for each of the cells in the Result column to support access from your scripting efforts.
- Incorporate CSS styling compatible with the themes of your own personal web site. You'll eventually mount this page on your site.
- Question 1. Add an onclick event function for Question 1 that places the correct result (Yes or No) in the corresponding cell of the Result column.
- Question 2. Develop the contents of row 2 that determines whether `y` is an integer.
- Complete the JavaScript for each of the remaining nine questions, testing your input thoroughly.
- Add four more interesting math questions appropriate for a Grade 10 math audience making creative use of ASCIIMathML capabilities and the resources of JavaScript's Math Object.
Decisions 1: The if Statement. ACES Pizzeria has decided to expand its menu to include Medium, X-Large, and Party sizes in addition to its Small and Large offerings. It also offers three payment options.
Task.
- Modify your ACESPizza.html page to have it appear similar to the image to the right.
- Add unique id fields to all 10 radio elements.
- In addition to the function $(id) introduced last week, implement three new functions as indicated below. This will make the code more compact.
// Returns the size of the requested pizza, as a String
function getSize(){
}
// Returns the means of conveyance of the pizza, as a String
function getConveyance(){
}
// Returns the means of payment for the pizza, as a String
function getPayment(){
}
|
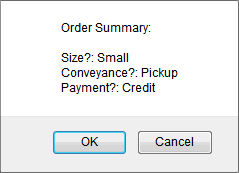 |
- Implement the bodies of the new functions and adjust the Order Summary confirmation.
- Review the HTML DOM Checkbox Object, then Try it yourself ».
- Return to ACESPizza.html. Between the Size and Conveyance fieldsets, add a Toppings fieldset. Within the set add three HTML DOM Checkbox objects for a random trio of choices. Modify your Order Summary to also identify the user's choices for toppings.
Boolean: Radio Buttons checked Property
ACES Pizzeria is a boutique operation that offers only Small or Large pizzas for either pickup or delivery and they've asked you to create a simple web form to provide online ordering support.
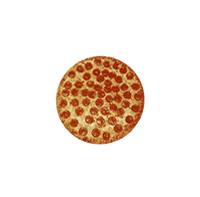 |
|
- Copy Shell.html to ACESPizza.html
- On the page, provide users with two sets of mutually exclusive radio buttons; one for either small or large size pizzas and one for either pickup or delivery. Set the defaults to small and pickup.
- Add a button with the label Place Order that, when clicked, offers the user a confirm box summarizing the users selections (see above, right).
- Click on the image of the small pizza above to identify its URL. Without downloading the image, place an <img> tag on your page that references the identical URL.
- When the user selects the size of the pizza, the image should change accordingly. Take care to secure the position of the image so that when it changes, the position of all page elements remains stable. To reference the image of a large pizza simply replace Small with Large in the URL.
The Number Object.
Read Wikipedia's description of Body-Mass Index (BMI).
- Copy Shell.html to BMI.html
- BMI.html should offer the user inputs to permit entry of the required data for a BMI calculation, a button to trigger the calculation of the value of the index, and a complete summary in the form of an alert box. Rather than just get an answer, I encourage you to use your HTML/CSS/JavaScript skills to create a work of art.
- Complete this exercise for homework and have it ready for the Randomizer next class.
Types. Save Shell.html as Types.html. In the <head> script, develop statements to accomplish the following tasks and use the console.log() function to display results to your browser's JavaScript Console Window where requested.
- Display the number 5.
- Display the type of data that 5 is an example of
- Declare a variable, x.
- Display x
- Assign the value 5 to the variable x and display the variable
- Display the type of x
- Display the type of data that "RSGC" is an example of
- Assign "RSGC" to x
- Display x
- Display the type of x
Use the console.log() function with String substitution techniques to display well-formatted results for each of the previous exercises.