Array Exercises (In class). Create a project called ArrayTest and
add statements to confirm each of the following tasks.
- Create a class, Arrays, that constructs an array of a user-defined
size and populates it with random 3-digit integers.
- Add a toString() method to your class to return a String equivalent of the
elements of the array,
- Provide an accessor method that returns the minimum value of
the array.
- Provide an accessor method that returns the maximum value of the array.
- Provide an accessor method that returns the average (arithmetic mean) of
the elements in the array.

- Provide an accessor method that returns the standard deviation of the elements in the array.
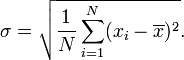
- Provide the method, public void
Shuffle() that shuffles the elements of the
array.
- Provide the method, public boolean
contains(int n) that returns true if
n is an element of the array, false otherwise.
- Provide the method, public boolean containsDuplicates() that returns true if the array contains any duplicates, false otherwise.
Matrix (2D Array) Exercises
- Instantiate a 2D int matrix, matA,
having 3 rows and 3 columns and populate it with random single-digit
integers.
- Instantiate another 3 by 3 2D int matrix, matB,
and initialize its contents to a known magic square.
- Write and test the method, display(int[][]
m), that displays the matrix
argument in rectangular form.
- Write and test the method, isMagicSquare(int[][]
m), that returns true if
the argument is a magic
square, false otherwise.